|
Matplotlib によるテキストのプロパティとそのレイアウトの制御
matplotlib.text.Text インスタンスには、テキストコマンドに対するキーワード引数( title() 、xlabel() 、text() など)を使用して設定できるさまざまなプロパティがあります。
Property |
Value Type |
alpha
|
float
|
backgroundcolor
|
any matplotlib color
|
bbox
|
Rectangle prop dict plus key 'pad' which is a pad in points
|
clip_box
|
a matplotlib.transform.Bbox instance
|
clip_on
|
bool
|
clip_path
|
a Path instance and a Transform instance, a Patch
|
color
|
any matplotlib color
|
family
|
[ 'serif' | 'sans-serif' | 'cursive' | 'fantasy' | 'monospace' ]
|
fontproperties
|
a FontProperties instance
|
horizontalalignment or ha
|
[ 'center' | 'right' | 'left' ]
|
label
|
any string
|
linespacing
|
float
|
multialignment
|
['left' | 'right' | 'center' ]
|
name or fontname
|
string e.g., ['Sans' | 'Courier' | 'Helvetica' ...]
|
picker
|
[None|float|boolean|callable]
|
position
|
(x, y)
|
rotation
|
[ angle in degrees | 'vertical' | 'horizontal' ]
|
size or fontsize
|
[ size in points | relative size, e.g., 'smaller', 'x-large' ]
|
style or fontstyle
|
[ 'normal' | 'italic' | 'oblique' ]
|
text
|
string or anything printable with '%s' conversion
|
transform
|
a Transform instance
|
variant
|
[ 'normal' | 'small-caps' ]
|
verticalalignment or va
|
[ 'center' | 'top' | 'bottom' | 'baseline' ]
|
visible
|
bool
|
weight or fontweight
|
[ 'normal' | 'bold' | 'heavy' | 'light' | 'ultrabold' | 'ultralight']
|
x
|
float
|
y
|
float
|
zorder
|
any number
|
整列引数 horizontalalignment、verticalalignment、multialignment を使ってテキストをレイアウトすることができます。 horizontalalignment は、テキストのx位置引数がテキスト境界ボックスの左、中央、または右を示すかどうかを制御します。 verticalalignment は、テキストのy位置引数がテキスト境界ボックスの下部、中央、または上部を示すかどうかを制御します。 multialignment は、改行で区切られた文字列の場合のみ、異なる行を左揃え、中央揃え、または右揃えにするかどうかを制御します。 次に、text() コマンドを使用してさまざまな配置の可能性を示す例を示します。 コード全体で transform = ax.transAxes を使用すると、軸の左下を0,0、右上を1,1とした座標が軸の境界ボックスに相対的に与えられます。
import matplotlib.pyplot as plt
import matplotlib.patches as patches
# build a rectangle in axes coords
left, width = .25, .5
bottom, height = .25, .5
right = left + width
top = bottom + height
fig = plt.figure()
ax = fig.add_axes([0, 0, 1, 1])
# axes coordinates are 0,0 is bottom left and 1,1 is upper right
p = patches.Rectangle(
(left, bottom), width, height,
fill=False, transform=ax.transAxes, clip_on=False
)
ax.add_patch(p)
ax.text(left, bottom, 'left top',
horizontalalignment='left',
verticalalignment='top',
transform=ax.transAxes)
ax.text(left, bottom, 'left bottom',
horizontalalignment='left',
verticalalignment='bottom',
transform=ax.transAxes)
ax.text(right, top, 'right bottom',
horizontalalignment='right',
verticalalignment='bottom',
transform=ax.transAxes)
ax.text(right, top, 'right top',
horizontalalignment='right',
verticalalignment='top',
transform=ax.transAxes)
ax.text(right, bottom, 'center top',
horizontalalignment='center',
verticalalignment='top',
transform=ax.transAxes)
ax.text(left, 0.5*(bottom+top), 'right center',
horizontalalignment='right',
verticalalignment='center',
rotation='vertical',
transform=ax.transAxes)
ax.text(left, 0.5*(bottom+top), 'left center',
horizontalalignment='left',
verticalalignment='center',
rotation='vertical',
transform=ax.transAxes)
ax.text(0.5*(left+right), 0.5*(bottom+top), 'middle',
horizontalalignment='center',
verticalalignment='center',
fontsize=20, color='red',
transform=ax.transAxes)
ax.text(right, 0.5*(bottom+top), 'centered',
horizontalalignment='center',
verticalalignment='center',
rotation='vertical',
transform=ax.transAxes)
ax.text(left, top, 'rotated\nwith newlines',
horizontalalignment='center',
verticalalignment='center',
rotation=45,
transform=ax.transAxes)
ax.set_axis_off()
plt.show()
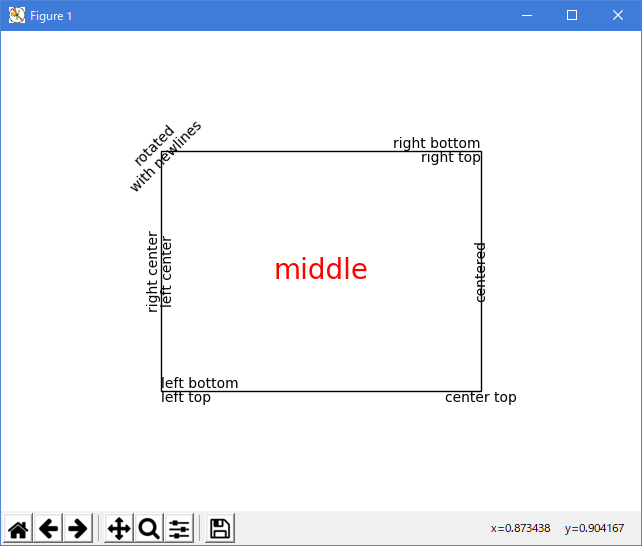
Top
|